This article is currently in the process of being translated into Japanese (~84% done).
Implementing a custom WPF Command
前の章では、すでにWPFに定義されているコマンドの様々な使い方を見てきましたが、もちろんあなたのオリジナルコマンドも同様に定義することが出来ます。それはとても簡単で、一度定義すればWPFの組み込みコマンドと同じ様に使うことが出来ます。
オリジナルのコマンドの実装する最も簡単な方法は、コマンドを含む static クラスを作ることです。各コマンドはクラスのスタティックフィールドとして加え、アプリケーションから使えるようにします。WPFは奇妙な理由で Exit/Quit コマンドが実装されていないので、我々のコマンドサンプルにそれを実装することにします。それはこの様になります。
<Window x:Class="WpfTutorialSamples.Commands.CustomCommandSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:self="clr-namespace:WpfTutorialSamples.Commands"
Title="CustomCommandSample" Height="150" Width="200">
<Window.CommandBindings>
<CommandBinding Command="self:CustomCommands.Exit" CanExecute="ExitCommand_CanExecute" Executed="ExitCommand_Executed" />
</Window.CommandBindings>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Menu>
<MenuItem Header="File">
<MenuItem Command="self:CustomCommands.Exit" />
</MenuItem>
</Menu>
<StackPanel Grid.Row="1" HorizontalAlignment="Center" VerticalAlignment="Center">
<Button Command="self:CustomCommands.Exit">Exit</Button>
</StackPanel>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
using System.Windows.Input;
namespace WpfTutorialSamples.Commands
{
public partial class CustomCommandSample : Window
{
public CustomCommandSample()
{
InitializeComponent();
}
private void ExitCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
e.CanExecute = true;
}
private void ExitCommand_Executed(object sender, ExecutedRoutedEventArgs e)
{
Application.Current.Shutdown();
}
}
public static class CustomCommands
{
public static readonly RoutedUICommand Exit = new RoutedUICommand
(
"Exit",
"Exit",
typeof(CustomCommands),
new InputGestureCollection()
{
new KeyGesture(Key.F4, ModifierKeys.Alt)
}
);
//Define more commands here, just like the one above
}
}
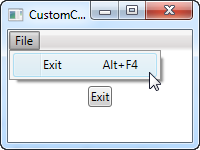
マークアップにメニューとボタンから成る簡単なインターフェースを定義しました。これらはどちらも新しい Exit カスタムコマンドを使っています。このコマンドはコードビハインドの CustomCommands クラスに定義されていて、コマンドの実行と実行可否判定に使うイベントを割り当てた、ウィンドウの CommandBindings コレクションから参照されます。
これら全ては、前章のサンプルとちょうど同じですが、組み込みのコマンドではなく、自身のコードのコマンドという点だけが違っています。
コードビハインドではコマンドの2つのイベントの処理をします。一つのイベントは、いつでも exit/quit コマンドを実行できるようにするため、常にTRUEにします。もう一つのイベントはアプリケーションを終了する Shutdown メソッドを呼びます。とても単純です。
すでに説明したように、Exitコマンドを static な CustomCommands クラスのフィールドに実装しました。コマンドの定義とプロパティの割当の方法はいくつかありますが、コンストラクタに全て割り当てるコンパクトな手法を取りました(1行に書いてしまえばもっとコンパクトになりますが、読みやすさのために改行しています)。そのパラメータは、コマンドのテキストまたはラベル、コマンドの名前、オーナータイプ、そしてコマンドのデフォルトショートカット(Alt+F4)を定義する、InputGestureCollection です。
まとめ
カスタムWPFコマンドの実装は、組み込みのコマンドを使うのとほとんど同じぐらい簡単です。そして、アプリケーションの色々な目的にコマンドを使うことが出来ます。これで、この章のサンプルに示したように、異なる場所からの機能の再利用がとても簡単になります。